Why PHP is a Loosely Typed Language?
PHP is a dynamically typed language, which means that variable types are determined at runtime and the language allows for implicit type conversion.
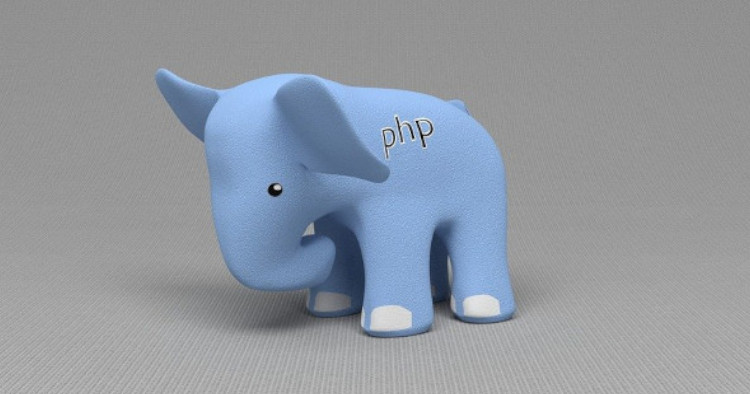
In a loosely typed language like PHP, variables are not bound to a specific data type during declaration or initialization.
Instead, the data type of a variable is inferred based on the value it holds at runtime.
This flexibility comes with both advantages and disadvantages.
Advantages of a Loosely Typed Language like PHP
Flexible and forgiving
PHP allows developers to perform operations without worrying about data types, leading to more straightforward and less verbose code.
This can be beneficial when working with different data types.
Easy to get started
The lack of strict type declarations in PHP can make it easier for beginners to pick up and start coding without having to deal with complex type-related issues.
Quick prototyping
Developers can quickly create and test ideas as they don’t need to explicitly define data types in their code.
Disadvantages of a Loosely Typed Language like PHP
Type-Related Bugs
The lack of strict typing can lead to unexpected behaviours and bugs in the code.
For instance, performing arithmetic operations on variables with mismatched types can result in unpredictable results.
Readability and Maintainability
Code can become harder to read and maintain as it may not be immediately apparent what data type a variable should hold at any given time.
Performance overhead
Dynamic typing can impose performance overhead since the interpreter must handle type conversions at runtime.
To address some of the disadvantages while maintaining flexibility, PHP introduced “strict typing” in PHP 7.
With strict typing, developers can opt to specify the data type of a function’s parameters and return values explicitly, making the code more predictable and less error-prone.
How to Enable Strict Typing in PHP?
Add the `declare` construct at the beginning of your script, before any other code
This feature was introduced in PHP 7.0 and allows you to enforce strict typing for function parameters and return values within the scope of the script.
<?php
declare(strict_types=1);
?>
The `strict_types=1` declaration tells PHP to enable strict typing for the entire script.
When you set `strict_types` to 1, PHP will enforce strict type checking for function arguments and return values.
Once strict typing is enabled, PHP will enforce the following rules:
- Function arguments must match their declared type exactly.
- For example, if a function parameter is declared as `int`, it must receive an integer value, and not allow implicit type conversions.
- Return types must match the declared type exactly.
- If a function is declared to return a `string`, it must return a string value, and not allow implicit type conversions.
- If strict typing is enabled and a type mismatch occurs, PHP will throw a `TypeError` at runtime.
Keep in mind that strict typing only applies within the scope of the file where it is declared.
If you include other PHP files or use code from external libraries that don’t have strict typing enabled, the strict typing rules won’t affect those parts of the code.
Enabling strict typing is considered a good practice as it helps catch type-related errors early in the development process, leading to more robust and maintainable code.