How to Integrate ChatGPT API in PHP?
Discover step-by-step instructions on integrating the ChatGPT API in PHP effortlessly. Enhance your website with AI-powered chat capabilities using this comprehensive guide.
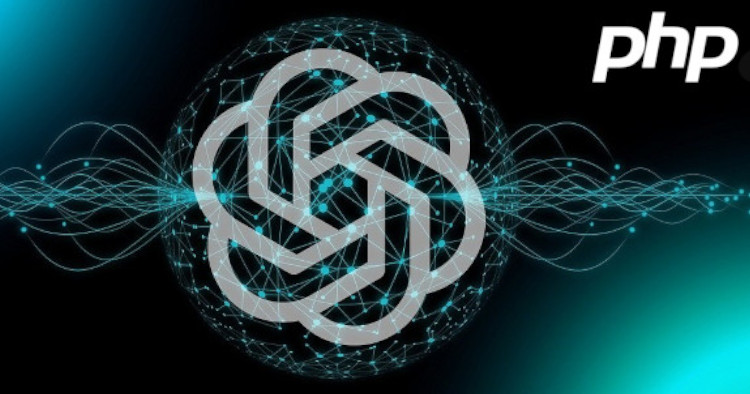
Step by Step Tutorial:
Setting API Key and Endpoint
$apiKey = 'your_api_key';
$endpoint = 'https://api.openai.com/v1/chat/completions';
First and foremost, you need to replace `your_api_key` with your actual OpenAI API key.
The `$endpoint` variable represents the URL where you will send the API request.
Setting Headers
$headers = array(
'Content-Type: application/json',
'Authorization: Bearer ' . $apiKey
);
Here, the `$headers` array contains two elements.
The first element sets the content type as JSON, and the second element includes the authorization header with your API Key.
Setting Data Payload
$data = array(
'messages' => array(
array('role' => 'system', 'content' => 'You are a helpful assistant.'),
array('role' => 'user', 'content' => 'What is the weather like today?')
)
);
$jsonData = json_encode($data);
The `$data` array represents the conversation passed to the API.
It contains two messages; >a system message and a user message.
You can modify this section to customize the conversation as needed.
Then we convert the `$data` array into a JSON-encoded string, as the API expects the payload to be in JSON format.
PHP cURL Request
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
The `curl_init()` function initializes a cURL session that will be used to send the API request.
Then we set various options for the cURL session to specify the URL, set the request method to POST, pass the JSON data as the request body, set the headers, and enable returning the response as a string.
The `curl_exec()` function sends the HTTP request to the API endpoint and returns the response.
Parsing the Response JSON
$result = json_decode($response, true);
The `json_decode()` function converts the response JSON string into a PHP associative array.
The resulting array is stored in the `$result` variable.
Accessing the Generated Message
$reply = $result['choices'][0]['message']['content'];
This line extracts the content of the generated message from the `$result` array.
It assumes that the response contains at least one choice, and it accesses the `content` field of the message.
Outputting the Reply
echo $reply;
Finally, the generated reply is displayed on the screen using the `echo` statement.
Make sure to replace `your_api_key` with your actual OpenAI API key before running the full code below.
Full Code
<?php
// Set your API key and the endpoint URL
$apiKey = 'your_api_key';
$endpoint = 'https://api.openai.com/v1/chat/completions';
// Set the headers
$headers = array(
'Content-Type: application/json',
'Authorization: Bearer ' . $apiKey
);
// Set the data payload
$data = array(
'messages' => array(
array('role' => 'system', 'content' => 'You are a helpful assistant.'),
array('role' => 'user', 'content' => 'What is the weather like today?')
)
);
// Convert the data to JSON
$jsonData = json_encode($data);
// Initialize cURL session
$ch = curl_init();
// Set the cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $jsonData);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Parse the response JSON
$result = json_decode($response, true);
// Access the generated message
$reply = $result['choices'][0]['message']['content'];
// Output the reply
echo $reply;
?>