How to Disable Right Click on Your Website using Javascript?
If you want to disable the right-click context menu on your website, follow the simple steps in this post. You might have to disable developer tool shortcuts as well.
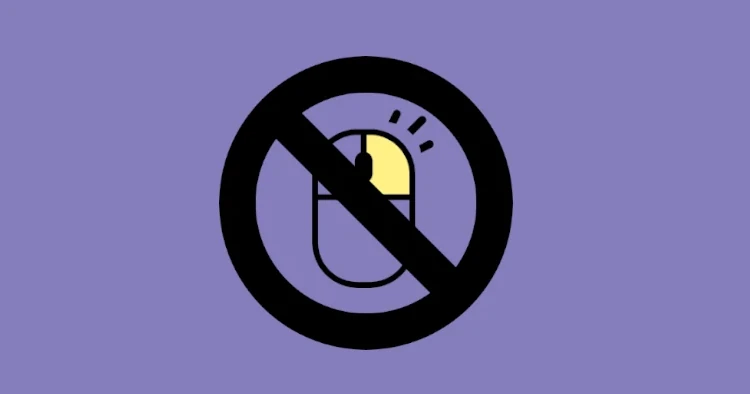
Note that if you want to disable the right-click menu on your website in order to prevent your users from viewing your source code or blocking them from downloading your images, there is no 100% way to do it.
A technical user can easily view your source code or download images from your site anyway. Although, by following the steps below, you can prevent some non-technical users from right clicking on your website.
Disable contextmenu Event
Whenever you right-click on your web page, a DOM event called “contextmenu” gets triggered. All you need to do is listen for this event and prevent the default browser functionality, as shown below.
document.querySelector("body").addEventListener("contextmenu", (event) => {
event.preventDefault();
});
You can simply call the preventDefault() method, and if you want, you can use the alert() method in javascript to throw an alert every time the user right-clicks.
Instead of disabling right-click on your entire page, you can just disable it in certain areas if you want.
document.getElementById("myDiv").addEventListener("contextmenu", (event) => {
event.preventDefault();
});
change the id from “myDiv” to whatever you have in your page.
Disable with Inline Javascript:
<div oncontextmenu="return false;">Right Click Disabled Here</div>
Disable Developer Tool Keyboard Shortcuts
In addition to the right-click menu, you should disable the shortcut keys for developer tools too. This will prevent some more users from accessing your code.
Users can still access developer tools from menus in their browser, but you can prevent a good amount of people who only use shortcuts and are too lazy to go through the browser menus.
document.addEventListener("keydown", (event) => {
var key = event.keyCode;
if (
key == 123 ||
(event.ctrlKey && event.shiftKey && key == 67) ||
(event.ctrlKey && event.shiftKey && key == 73)
) {
event.preventDefault();
}
});
So every time a user presses any key on their keyboard, we are just checking if they pressed F12 (key == 123), CTRL + SHIFT + C (key == 67), or CTRL + SHIFT + I (key == 73), If any of those shortcuts are triggered, we will simply call the preventDefault() method.