How to Create Simple Router in PHP?
Learn how to create a basic PHP router without using any bloated frameworks or other dependencies. You can further develop this into a full MVC framework.
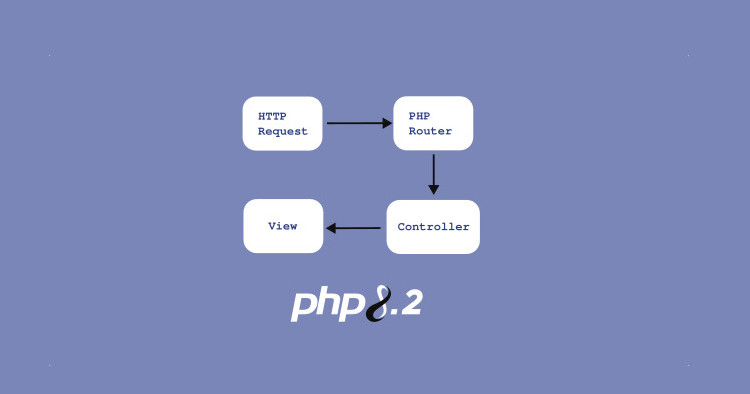
Folder Structure
You can set up a basic folder structure like the one below.
project-root/
├── app/
│ ├── controllers/
│ ├── models/
│ └── views/
├── public/
│ ├── css/
│ ├── js/
│ └── img/
└── .htaccess
└── index.php
Setup Apache .htaccess
Make sure your server has Apache mod_rewrite enabled.
Create a .htaccess file at the root of your web directory and paste this.
<IfModule mod_rewrite.c>
RewriteEngine On
# Allow all requests under public directory
RewriteRule ^public/ - [L]
# Route all other requests to index.php
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule (.*) index.php?route=$1 [L,QSA]
</IfModule>
So basically, we will let all the paths under public folder execute as they are without any processing.
And we will get all other paths and pass them as parameters to index.php.
Index File
Create index.php at the root of your web directory and paste the following content:
<?php
//define env variables
define('APP', dirname(__FILE__)."/app/");
// Get the route variable
$route = $_GET['route'];
$routeParts = explode("/", $route);
$base = $routeParts[0];
if($base == ""){ $base = "home"; }
$ctrl = APP."controllers/".$base.".php";
if(file_exists($ctrl)){
//load the controller
require_once($ctrl);
}else{
//Load the view directly
require_once(APP."views/404.php");
}
?>
We will get our path from the $_GET variable and split it by ”/”.
Then we will get the first part, and if it is empty, we will replace it with “home”.
We need to create each file under the app/controllers folder for each route, such as home.php, about.php, posts.php, and so on.
If the controller file is found, we will include it; otherwise, we will include “404.php” from the app/views folder to let users know that the requested path is not found on the server.
Controller
You can simply do all the logic and load the corresponding view file like this:
<?php
//Do all your logic here
//Then include the home view
require_once(APP."views/home.php");
?>
Or you can further process the route based on the HTTP request method like this:
<?php
$method = $_SERVER['REQUEST_METHOD'];
$id = $routeParts[1];
if($id != ""){
switch ($method) {
case 'GET':
echo "Single Post with the id ".$id;
break;
case 'POST':
echo "Update Post ".$id;
break;
case 'DELETE':
echo "Delete Post with the id ".$id;
break;
default:
echo "Not Found";
break;
}
}else{
switch ($method) {
case 'GET':
echo "All Posts";
break;
case 'POST':
echo "Create Post ";
break;
default:
echo "Not Found";
break;
}
}
?>
Obviously, you have to include the appropriate controller files instead of echo’ing from the above route.